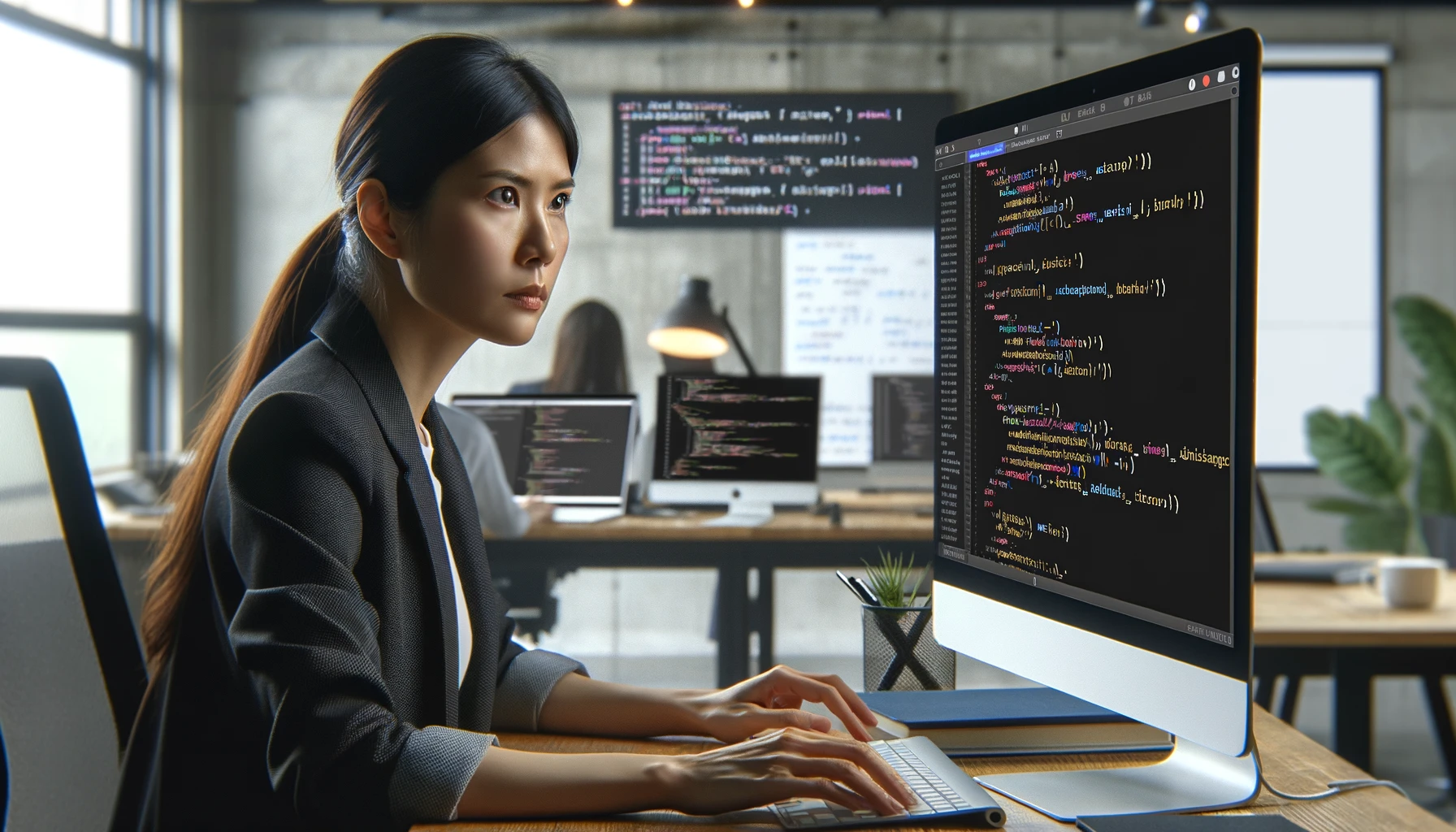
The Slice Misconception in PHP and Laravel
When dealing with PHP and the Laravel framework, a common pitfall arises from misunderstanding how the array_slice
function and the Collection::slice
method operate. This confusion often stems from the second argument in these functions being different than the related functions in other commonly used languages. Contrary to what some developers might expect from similar helpers in languages like Python and JavasScript, this argument represents the length of the slice, not the end index. This subtlety can lead to unexpected behaviors and bugs in your code.
The PHP array_slice
Function
PHP’s array_slice
function is a powerful tool for extracting a portion of an array. However, the key to using it effectively lies in understanding its parameters:
array_slice(array $array, int $offset, ?int $length = null, bool $preserve_keys = false): array
The $length
parameter here specifies how many elements you want in your slice, starting from the $offset
. Here’s a practical example:
$fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry'];
$slicedFruits = array_slice($fruits, 1, 3)
// Result: ['banana', 'cherry', 'date']
In this example, slicing starts from ‘banana’ (index 1) and includes three elements in total.
Laravel’s Collection slice
Method
Laravel, a popular PHP framework, introduces the Collection
class, which offers a more intuitive approach to handling arrays. The slice
method in Laravel’s collections is quite similar to PHP’s array_slice
function:
$collection->slice(int $offset, ?int $length = null): Illuminate\Support\Collection
Let’s consider an example:
use Illuminate\Support\Collection;
$collection = new Collection(['apple', 'banana', 'cherry', 'date', 'elderberry']);
$slicedCollection = $collection->slice(1, 3)->toArray();
// Result: ['banana', 'cherry', 'date']
The behavior is consistent with PHP’s array_slice
: we’re getting a length of 3 elements starting from index 1.
Comparison with Other Languages
It’s essential to understand how this differs from array slicing in other languages. For instance:
JavaScript: In JavaScript, the
slice
method uses start and end indices, where the end index is non-inclusive. This is different from PHP/Laravel, where the second argument is the length of the slice.let fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry']; let slicedFruits = fruits.slice(1, 4); // ['banana', 'cherry', 'date']
Python: Python’s slicing syntax is quite flexible and is an extension of the way that you index a list. In a simple case similar to the slicing described for other languages, you can use a colon in the index to separate a start and end index. Again, this differs from PHP’s approach of using the length of the slice.
fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry'] sliced_fruits = fruits[1:4] # ['banana', 'cherry', 'date']
Best Practices and Tips
Always double-check your slice parameters: Ensure you’re clear whether you’re specifying length or an end index.
Remember to check parameter names in IDEs: If you’re using an IDE like PHPStorm of VSCode, you can often hover over a function to see the parameter names and their order.
Consistency in team coding standards: If your team uses multiple languages, agree on a standard approach to slicing to reduce confusion.
Testing: Always test slices in your code to ensure they behave as expected, especially if your background is in a language with a different slicing approach.
Leverage Laravel collections: If you’re working within the Laravel framework, consider using collections for a more fluent and expressive syntax.
In conclusion, understanding the nuances of array slicing in PHP and Laravel is crucial for effective coding. By grasping the difference between the length of a slice and the end index, and by being aware of how this differs from other languages, you can avoid common pitfalls and write more robust, error-free code. Remember, clarity and consistency are your allies in the world of array manipulation.