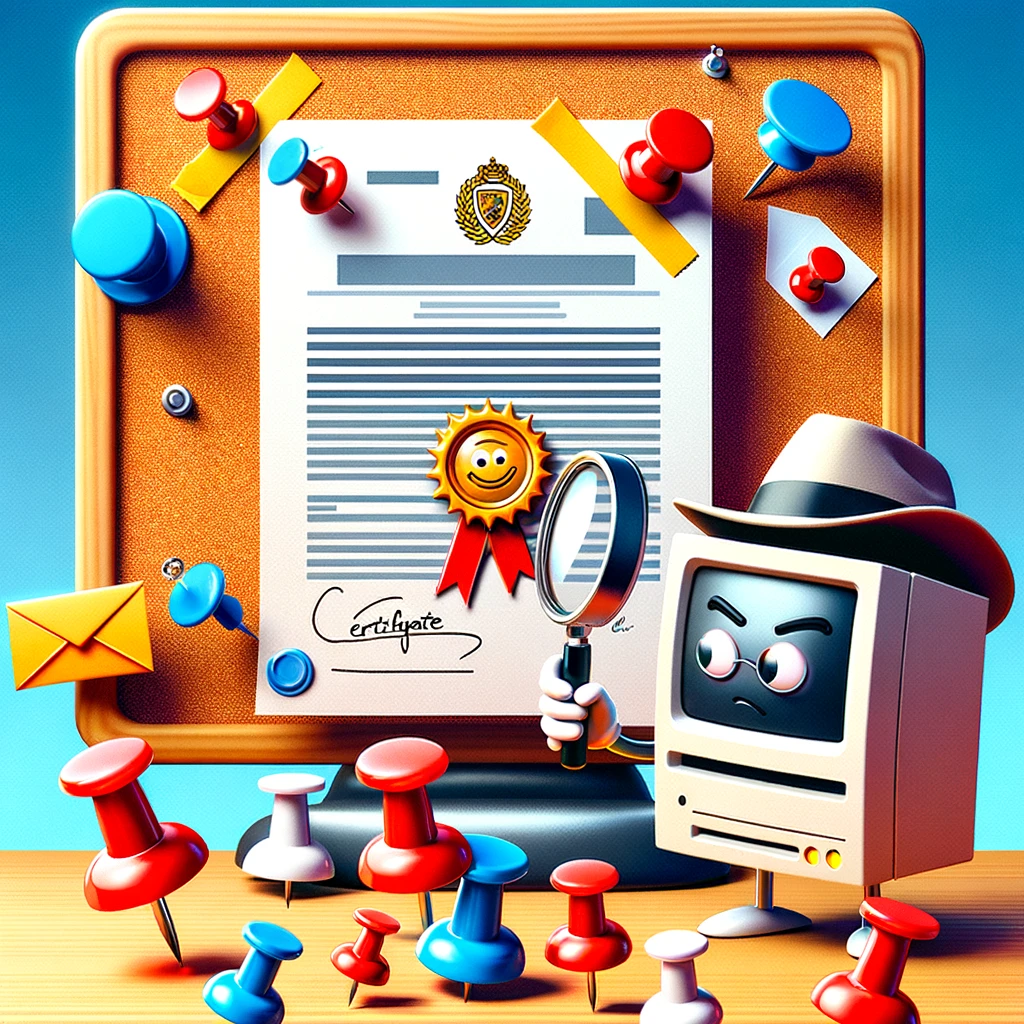
In the realm of cybersecurity, the protection of data in transit is paramount. Certificate pinning plays a crucial role in fortifying the security of software clients, acting as a defense mechanism against various attack vectors, including man-in-the-middle (MITM) attacks. This technique involves the software client validating the server’s certificate against a known copy of that certificate, ensuring that the connection is both secure and legitimate.
Understanding Basic Certificate Pinning
Certificate pinning, in its basic form, involves the client associating a host name with a server’s public certificate (which is derived from the public key). When the client establishes a connection with the server, it compares the server’s certificate or public key with the one it has. If there’s a match, the connection proceeds; if not, the connection is terminated.
This approach is particularly effective in preventing MITM attacks where an attacker might intercept the communication and present a forged certificate. By pinning the certificate, the client can ensure that it is communicating directly with the legitimate server.
However, basic pinning has its limitations. It can become problematic when the server’s certificate needs to be updated or replaced – an event which can occur due to expiration, revocation, or key compromise. In such cases, the application would need to be updated with the new certificate, which can be a significant logistical challenge.
Pinning Against the Certificate Chain
An advanced form of pinning is pinning against the certificate chain. This method involves the validation of one or more certificates in the server’s certificate chain, rather than the server’s certificate itself. Typically, this might be the root certificate or an intermediate certificate.
Pinning against the chain offers greater flexibility. Certificates higher up in the chain (like root or intermediate certificates) are less likely to change than the server’s own certificate. Therefore, pinning against these reduces the need for frequent application updates.
Scenarios Mitigated by Certificate Pinning
Certificate pinning is particularly effective in several scenarios:
Preventing MITM Attacks: As mentioned, pinning helps prevent attackers from intercepting communication by ensuring that the client only communicates with the server it trusts.
Protecting Against Compromised Certificate Authorities: In rare cases, a Certificate Authority (CA) might be compromised, leading to the issuance of fraudulent certificates. Pinning can mitigate this risk by ensuring that the client does not trust such certificates.
Enhancing Security in High-Risk Environments: In environments where security is of utmost importance, like banking or sensitive communication apps, pinning adds an extra layer of security.
Implementing Certificate Pinning in Client-Side Apps
Let’s look at a practical example of implementing certificate pinning in a client-side application. We’ll use a simple HTTP client in Python for demonstration purposes. Remember, the implementation will vary based on the programming language and the specific requirements of the application.
import requests
import hashlib
import hmac
# The expected SHA256 hash of the pinned certificate. Note this will
# need to be updated when the certificate changes.
PINNED_CERT_HASH = "33e4f5beda5033d26355a2fb200bb6d3a5d6e4d68be363eb5ebba5aeb420eb07"
def check_cert_pinning(cert):
# Extract the public key from the certificate
public_key = cert.public_key().public_bytes()
# Calculate the SHA256 hash of the public key
cert_hash = hashlib.sha256(public_key).hexdigest()
# Compare the calculated hash with the expected hash
# using a constant-time comparison function
return hmac.compare_digest(cert_hash, PINNED_CERT_HASH)
def main():
# URL of the server
url = "https://example.com"
# Send a GET request
response = requests.get(url, verify=False)
# Obtain the server's certificate
cert = response.raw.connection.sock.getpeercert(binary_form=True)
# Perform certificate pinning check
if check_cert_pinning(cert):
print("Certificate pinning successful.")
else:
print("Certificate pinning failed.")
if __name__ == "__main__":
main()
In this example, we compare the SHA256 hash of the public key in the server’s certificate with a pre-stored hash. If they match, the certificate is considered valid.
Best Practices and Considerations
When implementing certificate pinning, consider the following:
Fallback Mechanisms: Implement mechanisms to handle situations where the pinned certificate changes, such as a fallback list of acceptable certificates.
Certificate Chain Pinning: Consider pinning intermediate or root certificates to reduce maintenance overhead.
Security vs. Flexibility: Striking a balance between security and flexibility is crucial. Overly strict pinning might lead to issues with certificate renewals.
Regular Updates: Regularly update the application to reflect changes in the server’s certificates or the CA.
Error Handling: Implement robust error handling to manage situations where the pinning check fails.
Conclusion
Certificate pinning, when implemented correctly, significantly enhances the security of client-server communications. It’s a powerful tool against MITM attacks and compromised CAs, providing an additional layer of trust. However, it’s important to carefully plan and implement pinning strategies, considering the balance between security and practicality.
To delve deeper into this topic, explore resources like the OWASP guidelines, and always keep abreast of the latest security practices in the ever-evolving field of cybersecurity.