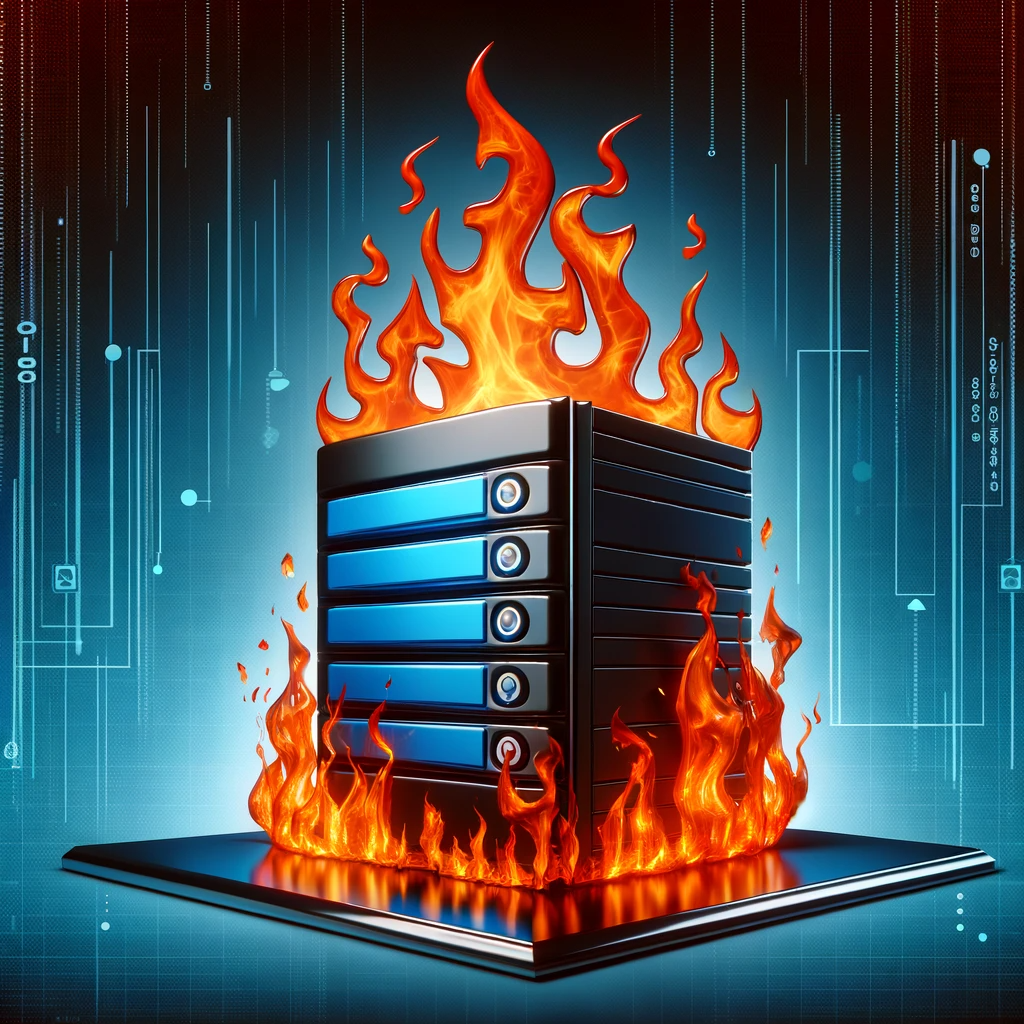
In web applications, performance issues can often stem from inefficient database queries. One such notorious problem is the N+1 query issue, which can significantly degrade the performance of an application. This post dives into understanding this issue and provides practical solutions to avoid it in ASP.NET, enhancing both the efficiency and scalability of your application.
Understanding the N+1 Query Problem
At its core, the N+1 query problem occurs when an application makes one query to retrieve a set of records (N) and then iteratively makes additional queries for each of these records (1 for each, thus N+1). This is a common issue in Object-Relational Mapping (ORM) tools like Entity Framework (EF) used in ASP.NET.
Consider an example: You have an Orders
table and a related OrderDetails
table in your database. You need to fetch all orders along with their order details. A naïve approach would be to fetch all orders and then loop through each order to fetch its details. This results in one query for the orders and N additional queries for the order details of each order.
var orders = context.Orders.ToList();
foreach (var order in orders)
{
var details = context.OrderDetails.Where(d => d.OrderId == order.Id).ToList();
// Do something with details
}
This approach can cause significant performance issues, especially with a large number of orders.
Recognizing the Symptoms
The N+1 problem might not be apparent during development, especially with small datasets. However, as the data grows, you might notice:
- Increased load times for pages displaying data from related tables.
- The SQL Profiler or similar tools showing a high number of similar queries with varying parameters.
- Overall sluggishness of the application when dealing with entity relationships.
Solutions to the N+1 Query Problem
1. Eager Loading
Eager loading is a technique where related entities are loaded from the database in the initial query. Entity Framework Core allows eager loading using the Include
method.
var orders = context.Orders.Include(o => o.OrderDetails).ToList();
This single query fetches all orders and their respective order details, drastically reducing the number of database calls.
2. Selective Loading
While eager loading is effective, it might sometimes lead to fetching more data than needed. Use projection to load only the necessary data.
var orderDetails = context.Orders.Select(o => new
{
o.Id,
o.Date,
Details = o.OrderDetails.Select(d => new { d.ProductName, d.Quantity })
}).ToList();
This approach ensures that only relevant data is loaded, maintaining efficiency.
3. Batch Queries
Batch queries are useful when you can’t avoid multiple database calls but want to optimize them. Tools like Entity Framework Plus offer batch query capabilities.
4. Utilizing AsNoTracking
When you’re only reading data and not updating it, use AsNoTracking
. This improves performance by not keeping track of changes in the retrieved entities.
var orders = context.Orders.AsNoTracking().ToList();
Best Practices
- Analyze Your Queries: Always analyze your SQL queries using tools like SQL Server Profiler or EF Core logging. This helps in identifying N+1 problems and other inefficiencies.
- Understand Your Data Relationships: Knowing how your entities relate helps in deciding when to use eager loading, lazy loading, or explicit loading.
- Beware of Over-Eager Loading: Eager loading everything can be as detrimental as the N+1 problem. Load only what’s necessary.
- Monitor Performance: Regularly monitor your application’s performance to catch any N+1 issues as your application evolves and the amount of data grows.
Conclusion
The N+1 query problem in ASP.NET applications can be a significant issue, but with the right strategies, it’s easily avoidable. By understanding your data and utilizing Entity Framework’s features effectively, you can ensure your application remains performant and scalable. Remember, every database call saved counts in the realm of web application performance.
Further Reading
By integrating these practices into your development process, you not only improve the performance of your ASP.NET applications but also enhance the overall user experience. Performance tuning, especially in database interactions, is an ongoing process. Stay vigilant, keep profiling your queries, and optimize as needed. Happy coding!