Segment is an analytics company that lets you consolidate your analytics in one place by routing one or more data sources to one or more destinations with ease. We’ll go over how to get started using Segment with React. This guide assumes that you’ve already created an account on Segment and have a React app up and running.
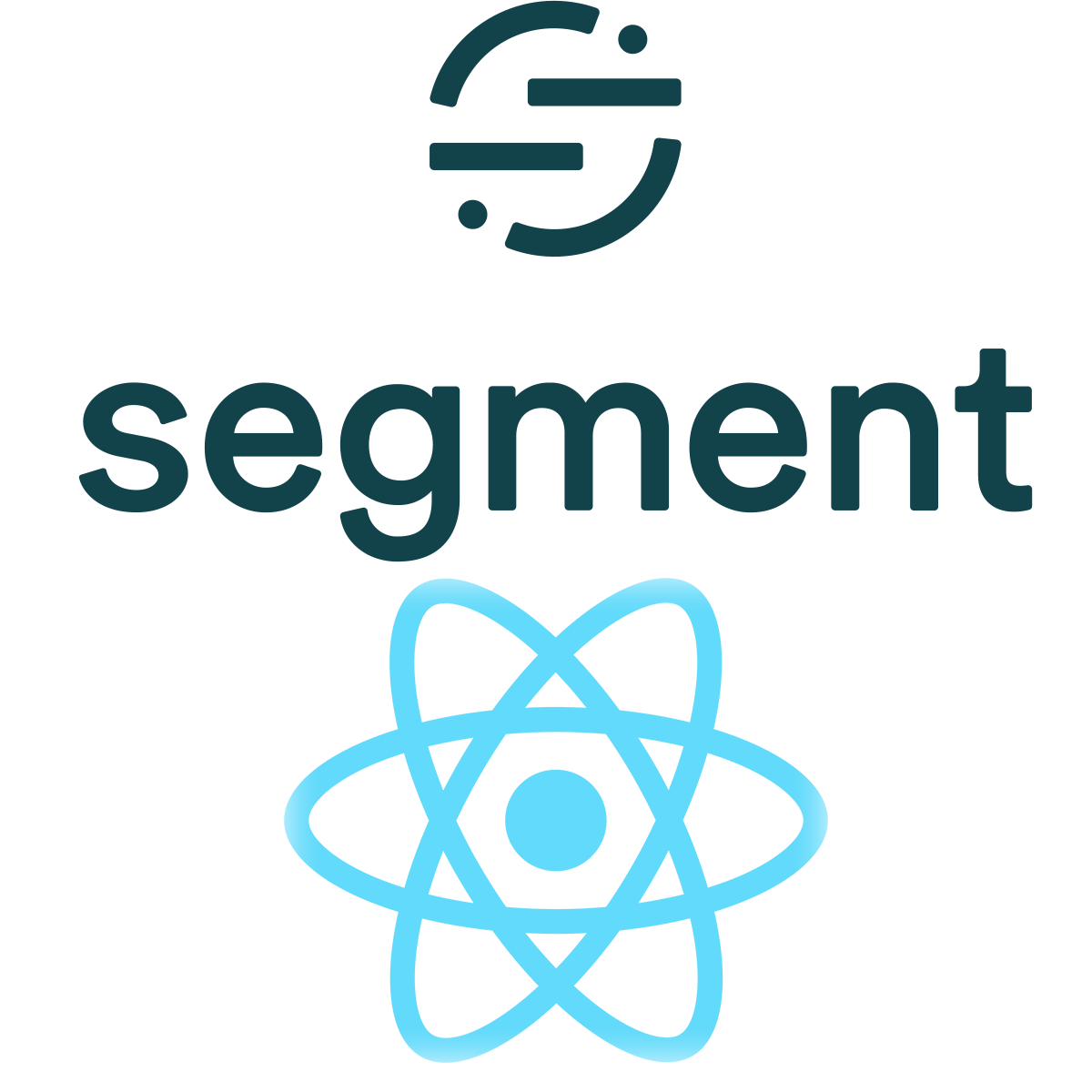
Getting started
Segment recommends that you add a JavaScript snippet to your HMTL page in order to integrate with their APIs. So if you are using Create React App, this would mean just adding your custom snippet from the Segment dash to your public/index.html
. Segment recommends this method of integration with JavaScript apps even though you may be used to installing packages from npm
. Adding this snippet will attach analytics
to your window, making window.analytics
or just analytics
globally available in your JavaScript.
Configuring for a React SPA
The Segment snippet is meant for a wide variety of JavaScript apps and does not assume that you are using a single-page app framework like React. There is one thing we need to do to get things to work for a React SPA using react-router for client-side routing. The default Segment snippet will call analytics.page()
automatically when a user initially loads a page which will log a page visit event for the current URL and title. However, when you use client-side routing and modify the URL using browser history, the analytics.page()
will not automatically continue to get called whenever the URL changes unless the user refreshes the page in their browser.
To resolve this issue, we need to hook up a listener to your browser history. You may already have a custom history object created using react-router. If not, details can be found here. You will then add a listener using something like this:
import { createBrowserHistory } from "history";
const history = createBrowserHistory();
let prevPath = null;
// listen and notify Segment of client-side page updates
history.listen((location) => {
if (location.pathname !== prevPath) {
prevPath = location.pathname;
analytics.page();
}
});
This works because the initial page event is already getting called by default from the Segment snippet, and then the subsequent changes will trigger this listen callback. The call to analytics.page()
is smart enough to grab the current URL at the time it’s called, so you don’t need to pass any parameters to it.
It’s important to note that the implementation above will only trigger updates if the URL path changes, but not if there are changes to query parameters. If you want events from query parameter changes as well, then you will want to include location.search
in the logic.
Identifying users
Segment also encourages calling analytics.identify()
once a user is identified after logging in. This is not required but allows you to associate events and page views with a specific user instead of just an anonymous user.
You likely already have some kind of callback after a user finishes logging into your app. You can add a call like this at that point.
const login = async (params) => {
const response = Api.post("login", params);
const user = response.data;
// notify segment of logged in user
analytics.identify(user.id, {
name: user.name,
email: user.email,
});
};
It is also recommended to call analytics.identify
whenever the user fields, like a name change. However if you have this hooked up to the login as shown above and the user has to login again fairly regularly it may be fine to just wait until the next login for this to get updated.
Tracking custom events
This should be enough to get you started with some basic usage data. However, you may want to track custom events in addition to page views if there are specific actions you want to track. To do this you will insert analytics.track
events any place you want to track an event. Common places include button clicks and component mounts. For example:
const SubmitButton = ({ onClick, value }) => {
const onClickButton = () => {
onClick();
analytics.track("submit_button.click", { value });
};
return <div onClick={onClickButton}>Submit</div>;
};
The case above would log a custom event named submit_button.click
and would pass extra parameters of the value
.
Hopefully this guide will be helpful in getting you started with Segment using React. Once you have your events getting logged correctly, you can hook up destinations within Segment to send your analytics to your preferred service, such as Google Analytics.